JWT Tokens or JSON Web Tokens are an industry-standard for token-based authentication and authorization. JWTs are digitally signed, which makes them secure and tamper-proof.
With Django, you can easily implement JWT-based authentication and authorization. By using JWTs, you can ensure that only authenticated users can access your application’s resources. JWTs also make it easy to share information securely between different parts of your application, such as between a front-end application and a back-end API.
Implementing JWT-based tokenization can significantly improve your application’s security and user experience. It can help prevent unauthorized access to your application’s resources, improve your application’s performance, and simplify the process of sharing information between different parts of your application.
What are JWT tokens?
JWT tokens a way of transmitting information between your app’s client and server, without the need for cookies or server-side sessions.
JSON Web Token (JWT) is an open standard (RFC 7519) that defines a compact and self-contained way for securely transmitting information between parties as a JSON object. This information can be verified and trusted because it is digitally signed. JWTs can be signed using a secret (with the HMAC algorithm) or a public/private key pair using RSA or ECDSA.
Source: https://jwt.io/introduction
JWT Components
The structure of a JWT token consists of three parts: the header, the payload, and the signature. Each part is encoded in Base64Url format and separated by periods (‘.’). Here is a breakdown of each component:
1) Header:
The header contains metadata about the JWT and consists of two parts: the token type (typ) and the signing algorithm (alg) used to secure the token. Common algorithms include HMAC, RSA, or ECDSA. The header is encoded using Base64Url.
Example Header:
{
"typ": "JWT",
"alg": "HS256"
}
Encoded Header:
eyJ0eXAiOiAiSldUIiwgImFsZyI6ICJIUzI1NiJ9
2) Payload:
The payload contains the claims, which are statements about the user or entity. Claims are classified into three types: registered claims, public claims, and private claims. Registered claims include predefined fields such as the issuer (iss), subject (sub), expiration time (exp), and audience (aud). Public claims are custom-defined claims specific to the application, while private claims are intended for use between parties that agree on their meaning. The payload is also encoded using Base64Url.
Example Payload:
{
"sub": "1234567890",
"name": "John Doe",
"admin": true
}
Encoded Payload:
eyJzdWIiOiAiMTIzNDU2Nzg5MCIsICJuYW1lIjogIkpvaG4gRG9lIiwgImFkbWluIjogdHJ1ZX0
3) Signature:
The signature is created by combining the encoded header, encoded payload, and a secret key or private key known only to the server. The signature verifies the integrity and authenticity of the token. The specific algorithm specified in the header is used to generate the signature. The signature is used to validate the contents of the token and ensure that it has not been tampered with.
Example Signature (using HMAC with SHA-256 algorithm):
HMACSHA256(
base64UrlEncode(header) + '.' +
base64UrlEncode(payload),
secret_key
)
Encoded Signature:
2WUAvX8JG53GFUN1d_YP0P6Mc9wG6lbyxgsOKXfG7fs
The final JWT token is formed by concatenating the encoded header, encoded payload, and encoded signature, separated by periods:
Final JWT Token:
eyJ0eXAiOiAiSldUIiwgImFsZyI6ICJIUzI1NiJ9.eyJzdWIiOiAiMTIzNDU2Nzg5MCIsICJuYW1lIjogIkpvaG4gRG9lIiwgImFkbWluIjogdHJ1ZX0.2WUAvX8JG53GFUN1d_YP0P6Mc9wG6lbyxgsOKXfG7fs
It’s important to note that while the JWT token structure is standardized, the specific values within the header and payload can vary depending on the implementation and application requirements.
You can use the JWT Debugger to see how a token is constructed and debugged.
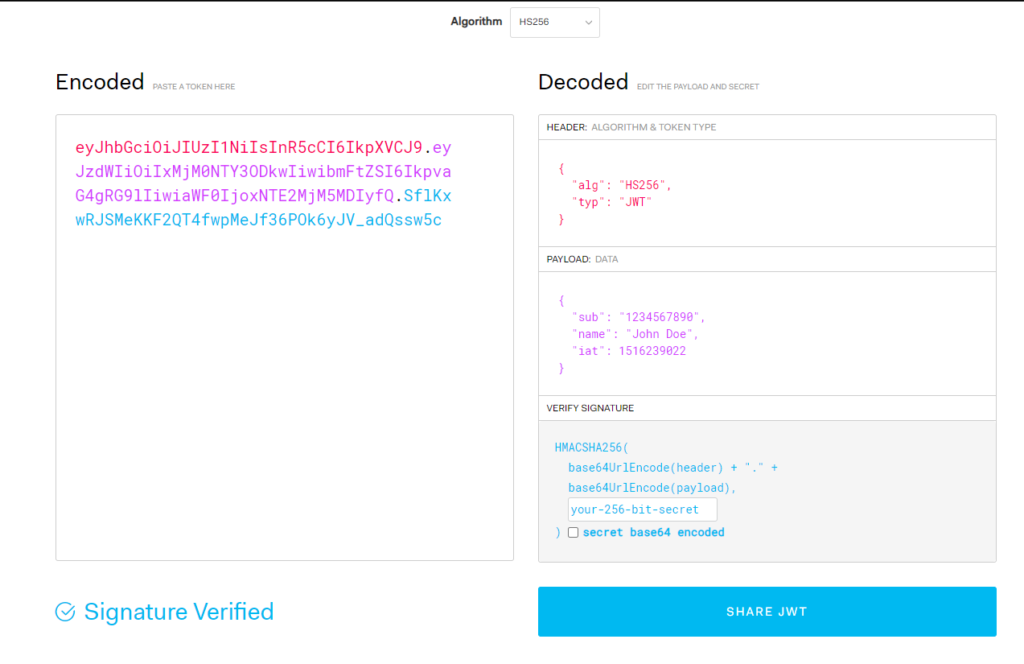
Benefits of using JWT tokens in Django Web Framework
There are many advantages of using JWT tokens in your Django Web Application. Some of them include:
JWT tokens are stateless
Due to the stateless nature of JWT tokens, the server does not need to keep track of the user’s session information. This makes it easier to scale your application and reduces the risk of session-related security vulnerabilities.
JWT tokens can be used across different domains
As JWT tokens can be used across different domains, it makes them useful for implementing Single Sign-On (SSO) solutions(a user can authenticate with one application and then access other applications without having to log in again).
JWT tokens are self-contained
JWT tokens can be verified without the need for additional communication with the server. This makes them ideal for use in microservices architectures, where each service can independently verify the authenticity of a user’s token.
JWT tokens are highly customizable.
The customizable nature of JWT tokens allows them to include all sorts of extra data beyond just authentication and authorization info. This is extremely useful when passing extra metadata or context between the client and server.
How can you use JWT tokens in Django Web Framework?
There are some great third-party libraries like django-rest-framework-simplejwt
that make it super easy to integrate JWT token authentication into your app.
Here’s an example of how you might use JWT tokens to authenticate a user in Django:
from rest_framework_simplejwt.tokens import RefreshToken
from django.contrib.auth.models import User
# create a new user
user = User.objects.create(username='testuser', password='testpassword')
# create a new refresh token for the user
refresh = RefreshToken.for_user(user)
# send the refresh token back to the client
return {'refresh': str(refresh)}
In the above example, we :
- Import the necessary dependencies :
RefreshToken
class from therest_framework_simplejwt.tokens
module, and theUser
model from Django’s built-indjango.contrib.auth.models
module.
- Create a new user with the
User.objects.create()
method. After passing in a username and password as arguments, a new user object is created in the database with the given username and password.
- Use the
RefreshToken.for_user()
method to create a new JWT token for the user we just created. This method generates a new refresh token that can be used to authenticate the user in future requests.
- Send the refresh token back to the client as a JSON response. The
str(refresh)
method call converts the token object to a string so that it can be sent over the network.
And here’s an example of how you might use JWT tokens to protect a view in Django:
from rest_framework_simplejwt.authentication import JWTAuthentication
from rest_framework.decorators import authentication_classes, permission_classes
from rest_framework.permissions import IsAuthenticated
@authentication_classes([JWTAuthentication])
@permission_classes([IsAuthenticated])
def my_view(request):
# this view requires authentication
Pass
In the above example, we’re using two decorators (function wrappers) to add JWT token authentication to a view :
@authentication_classes([JWTAuthentication])
decorator tells Django to use the JWTAuthentication
class to authenticate the user
@permission_classes([IsAuthenticated])
decorator tells Django to require that the user be authenticated before accessing the view.
By adding these decorators to the view function, we’re ensuring that only authenticated users can access the view.
Decorators are an important and advanced concept in python. To learn more about decorators read this article.