GitHub pages is a great way to showcase your projects online. With GitHub pages you can easily deploy and host your projects for free. You don’t have to worry about buying a seperate domain or major configuration changes.
Uploading vanilla HTML, CSS and JavaScript to Github Pages is super simple. However to deploy a React App to Github Pages is another thing all together!
In this artlicle, you’ll learn how to upload and deploy your React app to Github Pages.
Let’s talk about some prerequisites:
- You already have a react app ready to be deployed.
- You have some package manager like npm or yarn.
- You have an existing Github Account.
So let’s get started:
Step 1: Install the necessary dependencies
- Initialise a git repository in your project’s root folder. The root is the folder with the file
package.json
.
Step 2: Set up a Github Repository
- Login to your Github account.
- Create a new github repository in your github account. Call it
username.github.io
where username is your github usename. So if your github user name is matt, the repo should be calledmatt.github.io
.
Let’s now go back to our react project.
- Install the gh-pages package like so:
$ npm install gh-pages --save-dev
$npm react
Edit the package.json
file
- Open the
package.json
file in your text editor and add the following :
{
"name": "my-app",
"version": "0.1.0",
"homepage": "https://gitname.github.io/react-gh-pages", /* This line needs to be added */
"private": true,
In https://gitname.github.io/react-gh-pages
, gitname is your username. Here’s a visual Reference:
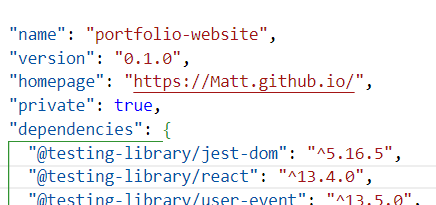
Under scripts add:
"scripts": {
"predeploy": "npm run build", /* This line needs to be added */
"deploy": "gh-pages -d build", /* This line needs to be added */
"start": "react-scripts start",
"build": "react-scripts build",
Here is a Visual Reference:
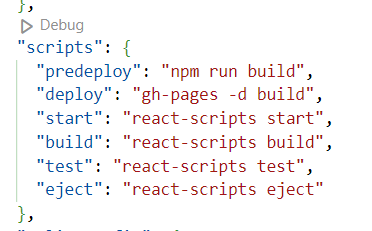
Add Remote and Deploy
- Add remote to the git repository of your project:
$ git remote add origin https://github.com/{username}/{repo-name}.git
- Run
npm run deploy
After these steps, your should have succesfully deployed your React Application on Github Pages.
It should live at https://username.github.io/
where username
is your Github Username. So if your Github Username is matt, your application should be at https://matt.github.io/
.
How to troubleShoot 404 Not found error:
If you are getting a 404 Not found error after following the above steps, the most common issue you might be having an issue with the Browser Router.
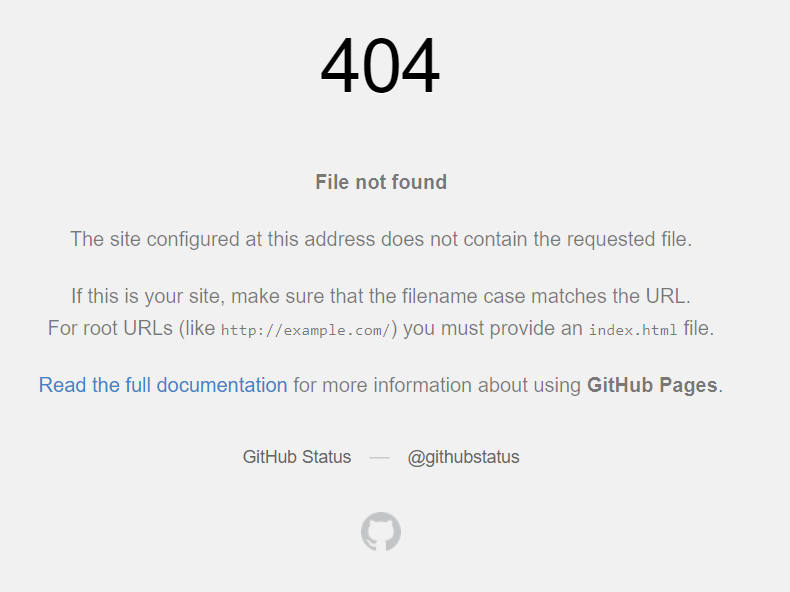
Browser Router is the most common router used to handle routing in React. But this router doesn’t seem to always work with Github pages. Instead, use Hash Router.
Follow this steps:
Install react-router-dom
npm install react-router-dom --save
Make changes to your index.js
Import HashRouter:
import { HashRouter } from "react-router-dom";
Change BrowserRouter
to HashRouter
Your index.js file should like this:
import React from "react";
import ReactDOM from "react-dom/client";
import "./index.css";
import App from "./App";
import { HashRouter } from "react-router-dom";
const root = ReactDOM.createRoot(document.getElementById("root"));
root.render(
<React.StrictMode>
<HashRouter>
<App />
</HashRouter>
</React.StrictMode>
);
Here is a visual reference:
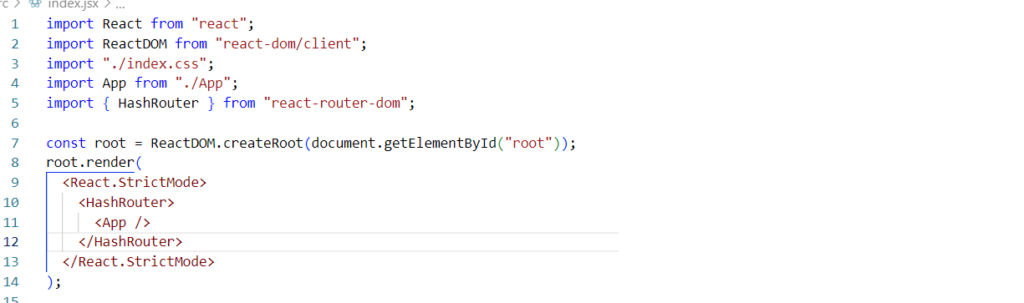
Deploy your application again using the deployment steps given above.
Happy Coding!