This Ultimate GIT Cheat Sheet has all the important GIT commands in one place, presented in an easy to navigate format. From fundamental operations like making commits and branching out to more intricate tasks such as resolving merge conflicts and fine-tuning commit histories; it’s your comprehensive guide to mastering GIT, covering a wide array of commands for all levels of use.
If you want a free PDF of this GIT Cheat Sheet, click here
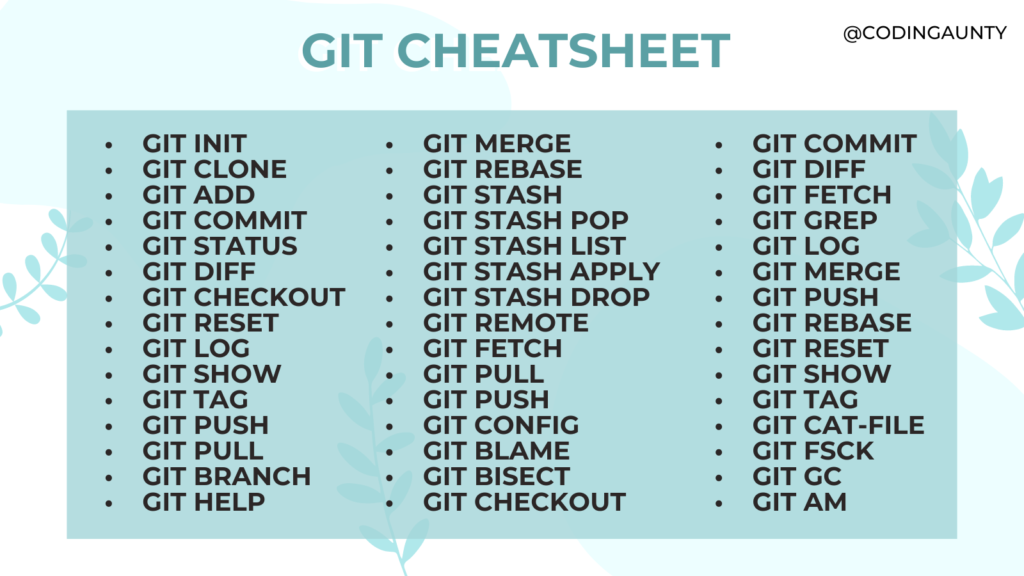
Table of Contents:
- What is Git?
- Installing Git
- Configuring Git
- Core Git Commands
- Git Branching Commands
- Git Stashing Commands
- Git Remote Commands
- Git Configuration Commands
- Git Plumbing Commands
- Git Porcelain
- Git Experimental
What is Git?
Git is a distributed version control system (VCS) used primarily for tracking changes in source code during software development.
It is an essential tool for modern software development, enabling efficient collaboration and management of code changes.
It lets teams of programmers work on the same project without stepping on each other’s toes. You can make changes, try new things, and easily merge it all together. It keeps a complete history of who did what and when. It also works offline and syncs up when you’re back online, making coding together or alone easy.
All in all Git is an essential tool for any Software Developer.
Installing Git
On Windows:
- Download Git: Visit the official Git website and download the latest version for Windows.
- Install Git: Run the downloaded executable file and follow the installation prompts. Make sure to choose the right settings for your needs during installation, especially the editor you plan to use with Git and the PATH environment.
- Verify Installation: Open the Command Prompt or Git Bash (installed with Git) and type
git --version
to verify the installation.
On macOS:
- Install with Homebrew (recommended): If you have Homebrew installed, simply open the Terminal and run brew install git.
- Install from the Official Git Website: You can also download the macOS Git installer from the official Git website.
- Verify Installation: Open the Terminal and type
git --version
to check the installation.
Configuring Git
After installing Git, you should configure your user information (name and email) as Git embeds this information into each commit you make.
- Open Terminal (on macOS/Linux) or Command Prompt/Git Bash (on Windows).
- Set Your Username: Run git config –global user.name “Your Name”
- Set Your Email Address: Run git config –global user.email “your_email@example.com”
- Check Configuration: Run git config –list to verify your settings.
Core Git Commands
These commands are fundamental for almost all Git operations:
git init
: Initialize a new Git repository.
git clone [url]
: Clone an existing repository.
git add [file]
: Stage changes for commit.
git commit -m "[commit message]"
: Commit changes to the repository.
git status
: Check the status of changes.
git branch
: Manage branches.
git checkout [branch-name]
: Switch branches.
git merge [branch]
: Merge branches.
git remote add [variable name] [Remote Server Link]
: Connect to a remote repository.
git push [variable name] [branch]
: Push changes to a remote repository.
git pull
: Fetch and merge changes from a remote repository.
git log
: Display commit logs.
git fetch [remote]
: Download changes from a remote repository.
git reset [file]
: Unstage changes.
git diff
: Show changes between commits, commit and working tree, etc.
git config --global user.name "[name]"
: Set a name for Git user.
git config --global user.email "[email address]":
Set an email for Git user.
Git Branching Commands
These commands are used for creating, managing, and manipulating branches in Git, providing essential tools for efficient version control and collaborative development.
git branch
: Lists all the local branches in the current repository.
git branch [branch-name]
: Creates a new branch.
git branch -d [branch-name]
: Deletes the specified branch. This is a “safe” operation in that Git prevents you from deleting the branch if it has unmerged changes.
git branch -D [branch-name]
: Force deletes the specified branch, even if it has unmerged changes.
git branch -m [old-branch-name] [new-branch-name]
: Renames a branch. If you are on the branch, you can omit the [old-branch-name].
git branch -a
: Lists all branches, local and remote.
git checkout [branch-name]
: Switches to the specified branch.
git checkout -b [branch-name]
: Creates a new branch and then checks it out.
git checkout -b [branch-name] [start-point]
: Creates a new branch starting from the specified commit, tag, or branch.
git merge [branch-name]
: Merges the specified branch into the current branch.
git merge --abort
: Aborts the merge process and tries to reconstruct the pre-merge state.
git rebase [base]
: Rebase the current branch onto the [base] branch.
git rebase --abort
: Aborts a rebase operation and returns to the original branch.
git rebase --continue
: Continues the rebase process after resolving conflicts.
git rebase -i [base]
: Performs an interactive rebase.
git branch --set-upstream-to=[remote/branch]
: Sets the upstream branch for the current branch.
git branch --unset-upstream
: Removes the upstream information for the current branch.
git cherry-pick [commit-hash]
: Applies the changes introduced by the specified commit on the current branch.
git branch -vv
: Lists all branches with more information including their upstream.
git branch --no-merged
: Lists branches that have not been merged.
git branch --merged
: Lists branches that have been merged.
git checkout --track [remote/branch]
: Creates a new branch that tracks a remote branch.
git checkout --orphan [branch-name]
: Creates a new orphan branch, which is a branch with no history.
git branch -f [branch-name] [start-point]
: Force moves a branch to a new start point.
git branch --show-current
: Displays the name of the current branch.
git branch [branch-name] [start-point]
: Creates a new branch at the specified start point.
Git Stashing Commands
These commands are used to temporarily store and manage uncommitted changes in your working directory.
git stash
: Temporarily stashes the changes you’ve made to your working directory. This allows you to save your current work state and revert to a clean working directory.
git stash pop
: Applies the changes from the most recently stashed state and then removes it from the stash list. If there are conflicts, the stash is not removed unless you add –index.
git stash list
: Lists all the stashed changesets. This command is useful for viewing all your stashed changes.
git stash apply
: Applies the changes from the most recently stashed state (or specified stash) to your working directory but keeps it in the stash list for possible later reuse.
git stash drop [stash@{}]
: Discards the most recent stash (or a specified stash if you include the index) from the list of stashed changes.
git stash clear
: Removes all the stashed states. This is helpful when you want to clean up and remove all saved stashes.
git stash create [message]
: Creates a stash with an optional message without removing the changes from the working directory.
git stash save [message]
: Similar to git stash, but allows you to provide a message for easy identification later. This command is deprecated in favor of git stash push.
git stash push [-u | --include-untracked]
: Creates a stash and includes untracked files if the -u or –include-untracked option is used.
- git stash branch [branch-name] [stash@{}]: Creates and checks out a new branch starting from the commit at which the stash was originally created, applying the stashed changes to the new working directory. If no stash is specified, the latest one is used.
git stash show [stash@{}]
: Displays the changes recorded in the stash as a diff between the stashed state and its original parent. Shows the latest stash if no stash is specified.
git stash apply --index
: Applies the changes and reinstates the index (staging area), so staged changes are still staged.
Git Remote Commands
These commands used for handling remote repositories in Git. They are essential for collaborating and managing code across different locations and teams.
git remote
: Lists all remote repositories.
git remote -v:
Lists all remote repositories along with their URLs.
git remote add [name] [url]
: Adds a new remote repository.
git remote remove [name]
: Removes a remote repository.
git remote rename [old-name] [new-name]
: Renames a remote repository.
git fetch [remote]
: Downloads new data from a remote repository but doesn’t integrate any of this new data into your working files.
git fetch --all
: Fetches changes from all configured remotes.
git pull [remote] [branch]
: Fetches changes from the remote repository and merges them into the current branch.
git push [remote] [branch]
: Uploads all local branch commits to the remote repository.
git push --all [remote]
: Pushes all branches to the remote repository.
git push [remote] --delete [branch]
: Deletes a branch on the remote repository.
git clone [url]
: Copies a remote repository into a new directory, automatically creating a local master branch.
git clone --mirror [url]
: Creates a bare repository that is a mirror of the remote repository.
git remote show [remote]
: Gives detailed information about a particular remote.
git remote update
: Fetches the most up-to-date objects from the remote repository.
git remote set-url [remote] [new-url]
: Changes an existing remote repository URL.
git remote set-head [remote] [-a | -d | [branch]]
: Sets or deletes the default branch (HEAD) of the remote.
git remote prune [remote]
: Deletes all stale remote-tracking branches under [remote].
git remote set-branches [remote] [branch]
: Changes the branches tracked by the remote.
git push --tags [remote]
: Pushes tags to the remote repository.
git ls-remote [remote]
: Lists all references, such as branches and tags, available in a remote repository.
git push [remote] [local-branch]:[remote-branch]
: Pushes a local branch to a specific branch on the remote repository.
git push [remote] :[remote-branch]
: Deletes a remote branch (equivalent to git push [remote] –delete [branch]).
Git Configuration Commands
These commands are used for configuring Git settings. These allow for customization at global, system, and local levels to optimize user experience and workflow efficiency.
git config --global [key] [value]
: Sets a configuration value at the global level, which is applicable across all repositories for the current user.
git config --system [key] [value]
: Sets a configuration value at the system level, which is applicable to all users on the machine.
git config --local [key] [value]
: Sets a configuration value at the local repository level.
git config --global --unset [key]
: Unsets a global configuration value.
git config --system --unset [key]
: Unsets a system-level configuration value.
git config --local --unset [key]
: Unsets a local repository configuration value.
git config --list
: Lists all the configuration settings.
git config --global --list
: Lists global configuration settings.
git config --system --list
: Lists system-level configuration settings.
git config --local --list
: Lists local repository configuration settings.
git config [key]
: Gets the value for a given key.
git config --global user.name "[name]"
: Sets the global username.
git config --global user.email "[email]"
: Sets the global email.
git config --global core.editor "[editor]"
: Sets the text editor to be used with Git commands.
git config --global alias.[alias-name] [git-command]
: Creates an alias for a Git command.
git config --global --edit
: Opens the global configuration file in a text editor for manual editing.
git config --system --edit
: Opens the system-level configuration file in a text editor for manual editing.
git config --local --edit
: Opens the local repository configuration file in a text editor for manual editing.
git config --global color.ui auto
: Enables automatic command line coloring for Git for easy reviewing.
git config --global core.autocrlf [true|false|input]
: Sets the handling of line endings in Git.
git config --global push.default [matching|simple|upstream|current]
: Sets the default mode for git push.
git config --global merge.conflictstyle [merge|diff3]
: Sets the style of conflict markers in merge conflicts.
git config --global pull.rebase false
: Configures the default behavior of git pull to merge.
git config --global rebase.autoStash true
: Enables automatic stashing before rebase operations.
git config --global credential.helper cache
: Enables temporary caching of credentials.
Git Plumbing Commands
Git plumbing commands are the low-level commands that provide the core functionality of Git. These commands are the foundational building blocks upon which the more user-friendly “porcelain” commands (like git commit
, git merge
, etc.) are built. Git’s lower-level plumbing commands, offering insights into the advanced and foundational operations that power Git’s internal mechanisms.
git cat-file [type] [object]
: Provides content or type and size information for repository objects.
git checkout-index [options] [--] [paths…]
: Copies files from the index to the working tree.
git commit-tree [tree] [-p parent] [-m message]
: Creates a new commit object from the provided tree object and log message and prints its SHA-1 hash.
git diff-tree [options] [<commit> | <tree>]
: Compares the content and mode of trees found via two tree objects.
git for-each-ref [options] [--] [pattern]
: Lists references in a local repository, optionally filtered by a pattern.
: Computes object ID and optionally creates a blob from a file.git hash-object [options] [--] <path>
git ls-files [options] [--] [path]
: Shows information about files in the index and the working tree.
git ls-remote [options] [<repository> [<refs>...]]
: Lists references in a remote repository.
git merge-tree [base-tree] [branch1-tree] [branch2-tree]
: Runs a three-way tree merge.
git read-tree [options]<tree-ish>
: Reads tree information into the index.
git rev-parse [options] [--] [args]
: Parses revision specifications, command line options, and file names.
git show-branch [--topics] [commit]
: Shows branches and their commits.
git show-ref [options] [--] [pattern]
: Lists references in a local repository.
git symbolic-ref [options] name [ref]
: Reads, modifies, or deletes symbolic refs.
git tag --list
: Lists tags in a repository.
git update-ref [options] <refname> <newvalue> [<oldvalue>]
: Updates the object name stored in a ref safely.
git mktree [--missing] [--batch] [--normalize]
: Reads standard input and creates a tree object.
git pack-objects [--stdout | -o <file> ] [--all-progress]
: Creates a packed archive of the objects in the repository.
git unpack-objects [--strict] [-n] [-q] [--recover] < <packfile>
: Unpacks objects from a packed archive.
git verify-pack [-v] <pack>…
: Validates the packed Git archive.
git write-tree [--missing-ok] [--prefix=/]
: Creates a tree object from the current index.
Git Porcelain
Git porcelain commands are the higher-level commands that provide a user-friendly interface for the majority of Git operations. These commands abstract the complexities of Git’s internal workings (handled by plumbing commands) into a more accessible and easy-to-use format.
git blame [file]
: Shows what revision and author last modified each line of a file.
git bisect:
Use binary search to find the commit that introduced a bug.
git grep
: Prints lines matching a pattern.
git reset [commit]
: Resets the current HEAD to the specified state.
git show [object]
: Shows various types of objects.
git tag [tagname] [commit]
: Creates, lists, deletes or verifies a tag object signed with GPG.
git rm [file]
: Removes files from the working tree and from the index.
git mv [file] [new file]
: Moves or renames a file, a directory, or a symlink.
git clone [url]
: Clones a repository into a new directory.
Git Experimental
Git experimental commands are those that are either in the experimental stage of development or are less commonly used, often for very specific purposes. They might not be as stable or widely documented as the standard Git commands. While these commands are not as commonly used as the standard Git commands, they provide specialized functionalities that can be crucial for certain advanced workflows. It’s important to use them with an understanding of their specific use cases and potential effects on your repository.
git annex
: Manages large files without storing them in the Git repository.
git am
: Applies a series of patches from a mailbox.
git cherry-pick --upstream
: Experimental command for upstream cherry-picking.
git describe
: Describes a commit using the most recent tag reachable from it.
git format-patch
: Formats and exports a series of commits as emailed patches.
git fsck
: Verifies the connectivity and validity of the objects in the database.
git gc
: Cleans up unnecessary files and optimizes the local repository.
git help
: Shows help for Git commands.
git log --merges
: Lists commit logs that are merges.
git log --oneline
: Displays the commit logs in a short, one-line format.
git log --pretty=
: Formats the log output using a variety of user-specified formats.
git log --short-commit
: Shows a shorter version of the commit ID.
git log --stat
: Shows stats (file changes, insertions, deletions) for the commit log.
git log --topo-order
: Orders commits in topological order.
git merge-ours
: Merges using the ‘ours’ strategy.
git merge-recursive
: Merges using the ‘recursive’ strategy.
git merge-subtree
: Merges using the ‘subtree’ strategy.
git mergetool
: Invokes a merge resolution tool.
git mktag
: Creates a tag object.
git mv
: Moves or renames a file, a directory, or a symlink.
git patch-id
: Generates patch identifiers.
git p4
: Synchronizes between Perforce and Git.
git prune
: Prunes all unreachable objects from the object database.
git pull --rebase
: Fetches from and rebases on top of another repository or a local branch.
git push --mirror
: Pushes a mirror of the local repository.
git push --tags
: Pushes tags to the remote repository.
git reflog
: Manages reflog information.
git replace
: Creates, lists, deletes, or verifies replace refs.
git reset --hard
: Resets the current HEAD to the specified state, discarding all changes.
git reset --mixed
: Resets the index but not the working tree.
git revert
: Reverts existing commits.
git rm
: Removes files from the working tree and the index.
git show-branch
: Shows branches and their commits.
git show-ref
: Lists references in a local repository.
git stash save
: Saves your local modifications away and reverts the working directory to match the HEAD commit.
git subtree
: Merges and splits subtrees from your project.
git tag --delete
: Deletes a tag.
git tag --force
: Replaces an existing tag.
git tag --sign
: Creates a signed tag.
git tag -f
: Forcefully creates or updates a tag.
git tag -l
: Lists tags.
git tag --verify
: Verifies a signed tag.
git unpack-file
: Creates a new file with the content of a blob object.
git update-index
: Registers file contents in the working tree to the index.
git verify-pack
: Validates packed Git archives.
git worktree
: Manages multiple working trees attached to the same repository.
Remember, mastering Git is a progressive journey. The more you use and experiment with these commands, the more proficient you’ll become. Keep this cheat sheet handy as a quick reference (Bookmark it) when working on your projects or when you need to recall a less frequently used command.
Here is a free PDF of this GIT Cheat Sheet:
Happy Coding!